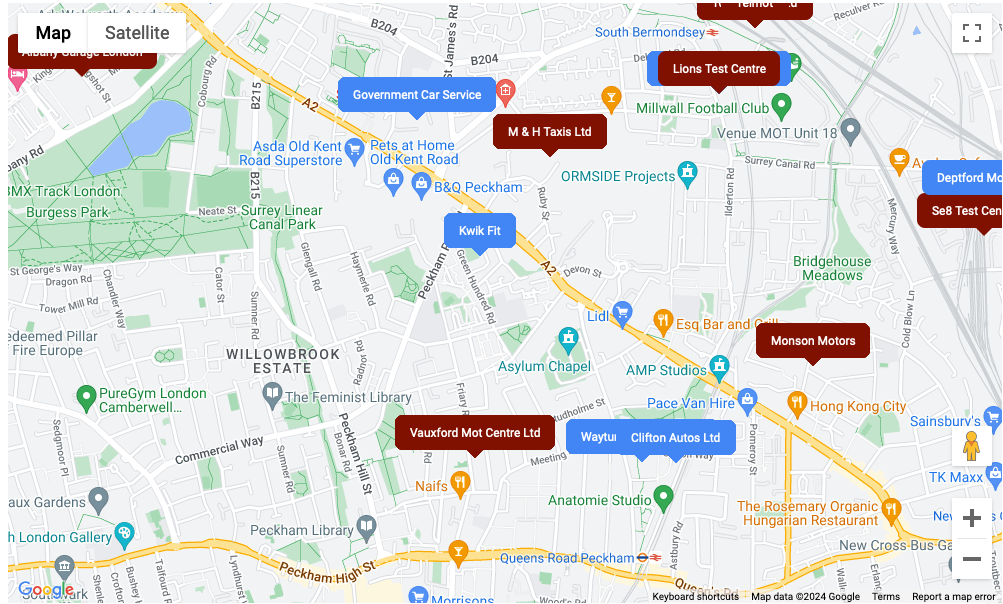
In today’s digital age, having an interactive map on your website can significantly enhance user experience, especially for businesses with multiple locations. Let’s explore how to integrate Google Maps JavaScript API with a custom database to create a powerful location-based search feature.
Setting Up Google Maps
First, you’ll need to set up a Google Maps JavaScript API key. Once you have your key, include the Google Maps script in your HTML:
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&libraries=places"></script>
Next, create a div element to hold your map:
<div id="map-canvas" class="zoom14"></div>
Initializing the Map
In your JavaScript file, initialize the map:
function initMap() {
const map = new google.maps.Map(document.getElementById("map-canvas"), {
zoom: 14,
center: { lat: 0, lng: 0 },
});
// More code will go here
}
Integrating with Custom Database
To populate the map with your company locations, you’ll need to fetch data from your custom database. This can be done using AJAX:
$.ajax({
url: 'get_locations.php',
method: 'GET',
success: function(response) {
const locations = JSON.parse(response);
locations.forEach(location => {
new google.maps.Marker({
position: { lat: location.lat, lng: location.lng },
map: map,
title: location.name
});
});
}
});
Implementing Distance-Based Search
To add a distance-based search, you can use the Google Maps Distance Matrix Service:
const service = new google.maps.DistanceMatrixService();
function searchNearby(origin, maxDistance) {
service.getDistanceMatrix(
{
origins: [origin],
destinations: locations.map(loc => new google.maps.LatLng(loc.lat, loc.lng)),
travelMode: 'DRIVING',
}, (response, status) => {
if (status === 'OK') {
const results = response.rows[0].elements;
results.forEach((result, index) => {
if (result.distance.value <= maxDistance) {
// Show this location on the map
}
});
}
}
);
}
By integrating Google Maps with your custom database, you can create a powerful tool for users to find your nearest locations. This not only improves user experience but also helps drive foot traffic to your physical stores.
Remember to style your map to match your brand and consider adding additional features like info windows for each location to provide more details to your users.